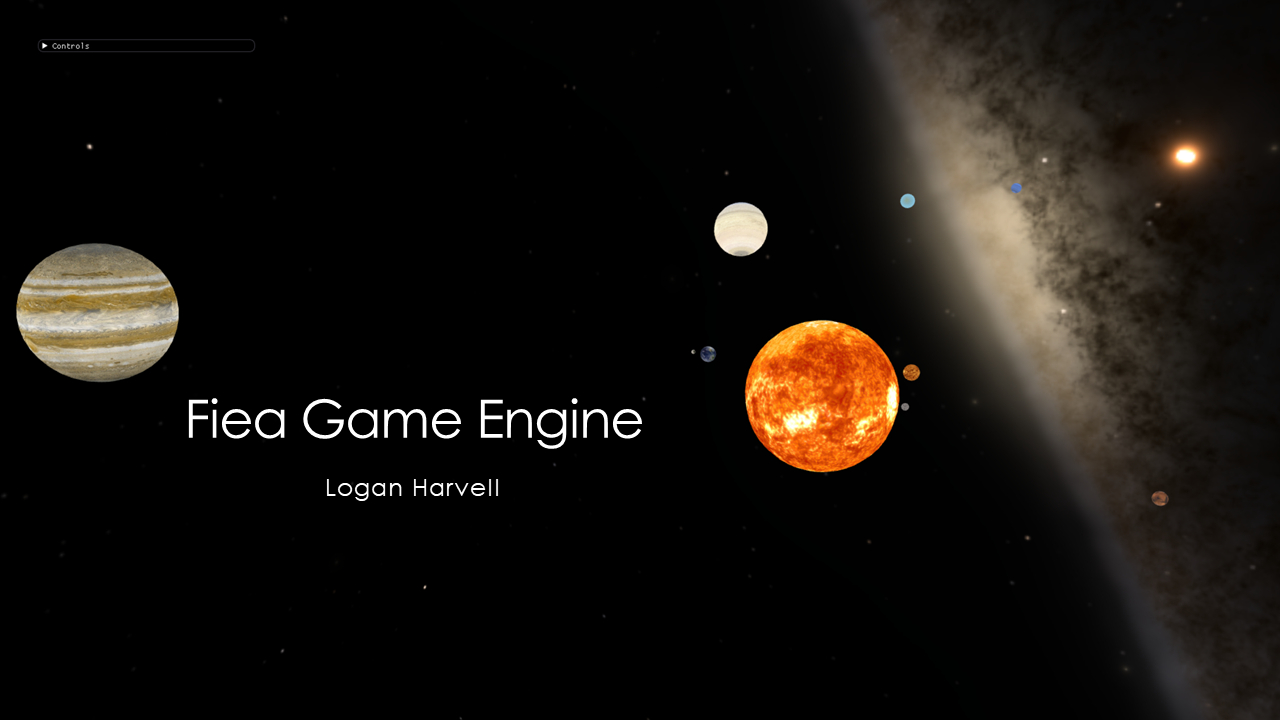
Fiea Game Engine
- 4 minsSummary
In January 2020, during the start of my spring semester, this project began as part of the curriculum in the programming track at UCF’s Florida Interactive Entertainment Academy. I was tasked with designing and implementing a game engine in C++ from the ground up. Starting with fundamental templated container classes mirroring the C++ standard library to a full property-centric runtime type reflection system and a multi-threaded event system.
Having always been interested in engine development and systems design, I tackled these tasks head on. Over the course of the semester, I learned a whole lot about engine development. However, there is infinitely more to learn, so I continue to develop this project as a means of exploring engine and systems development further. Explore the source code for the engine on GitHub.
As of May 2020, I have begun work on a rendering abstraction layer for the engine with the intention of enabling support for multiple rendering API. The abstraction layer will act as an interface for rendering functionality that could then be implemented by any rendering API, i.e. OpenGL, Vulkan, DirectX11, DirectX12, and Metal, as needed to best support the developed application and it’s target platforms. You can read more about this in the weekly developer diary blog series starting with the first post here.
Overview
If you would like to view the overview presentation slides for the Fiea Game Engine, click here.
Core
- Containers
SList
, a templated singly linked list class with support for forward iteratorsVector
, similar tostd::vector
with support for random access iteratorsHashMap
, similar tostd::unordered_map
with support for bi-directional iteratorsStack
, adaptor class forVector
, represents a basic stackDatum
, a vector class whose type can be determined at runtime through use of a discriminated union
- Runtime Type Reflection System
RTTI
, a custom base class for runtime type informationScope
, analogous to a property table, usingHashMap
,Vector
, andDatum
to store key-data pairsAttributed
, aScope
derived class that supports prescribed attributes (properties)TypeManager
, a singleton that manages type-attribute registration forAttributed
derived classesFactory
, a templated implementation of the abstract factory design pattern
- Generic Parser
- Utilizes the chain-of-responsibility pattern
- Combined with
Factory
, this enables the engine to be completely data driven - Handler for parsing JSON data into the reflection system, enabling JSON as a configuration language
- Events
IEventSubscriber
, acts as an interface for classes to observe an eventEventPublisher
, publishes an event to subscribersEvent
, subject that can be subscribed to byIEventSubscriber
instances- Derived from
EventPublisher
- Takes a generic type “payload” as a template parameter
- Manages the list of subscribers to the event
- Derived from
EventQueue
, manages the queue of events, firing an event as it expires
- Entity System
Entity
, anAttributed
derived class thinly wrappingScope
, represents a base level engine object- All engine classes that wish to be integrated into the core game loop derive from this class
- Supports composition through parent and child relationships with other entities
World
, a top levelEntity
class that manages a simulation, a.k.a. a game- Owns the
GameClock
and theWorldState
, which owns reference toGameTime
and theEventQueue
- Uses the above classes to manage the game loop
- Root
Entity
objects can be added/removed, analogous to loading/unloading a level
- Owns the
Reaction
, a generic implementation ofIEventSubscriber
integrated into the reflection system
- Math
Transform
, represents position and orientation transformations of an object in 3D space
Engine
- Entities
Actor
, anEntity
with aTransform
, supports hierarchical transforms
- Components
Model
, represents the physical attributes, can be loaded from a 3D modelAnimatorComponent
, manages playing animations
- Actions
- Several
Entity
derived classes that grant behavior to their parent class - Can be used for any behavior including data manipulation and control flow
- Used with the parser and reflection system to define behavior within JSON
- Several
Goals
After wrapping up the initial engine development towards the end of April 2020, the first major milestone for the engine was marked by its use in the recreation of the battle mode of Super Bomberman for the SNES.
The next goal of this project will be the culmination of the current work on a rendering abstraction layer for the engine. This is planned as a visual demonstration of a dynamic scene rendered using the rendering abstraction layer with an implementation both in OpenGL 4.6 and DirectX11 as a proof-of-concept.